class ProductClassShape[E <: Product, C <: Product] extends MappedScalaProductShape[FlatShapeLevel, Product, C, E, C]
A generic Product class shape that can be used to lift a class of plain Scala types to a class of lifted types. This allows the type to be used as a record type (like tuples and HLists) in the Lifted Embedding.
This can help with mapping tables >22 columns to classes, especially when using code generation. This can be used for Scala 2.11 case classes >22 fields.
Example:
def columnShape[T](implicit s: Shape[FlatShapeLevel, Column[T], T, Column[T]]) = s class C(val a: Int, val b: Option[String]) extends Product{ def canEqual(that: Any): Boolean = that.isInstanceOf[C] def productArity: Int = 2 def productElement(n: Int): Any = Seq(a, b)(n) } class LiftedC(val a: Column[Int], val b: Column[Option[String]]) extends Product{ def canEqual(that: Any): Boolean = that.isInstanceOf[LiftedC] def productArity: Int = 2 def productElement(n: Int): Any = Seq(a, b)(n) } implicit object cShape extends ProductClassShape( Seq(columnShape[Int], columnShape[Option[String]]), seq => new LiftedC(seq(0).asInstanceOf[Column[Int]], seq(1).asInstanceOf[Column[Option[String]]]), seq => new C(seq(0).asInstanceOf[Int], seq(1).asInstanceOf[Option[String]]) )
- Source
- Shape.scala
- Alphabetic
- By Inheritance
- ProductClassShape
- MappedScalaProductShape
- MappedProductShape
- ProductNodeShape
- Shape
- AnyRef
- Any
- by any2stringadd
- by StringFormat
- by Ensuring
- by ArrowAssoc
- Hide All
- Show All
- Public
- Protected
Instance Constructors
Type Members
Value Members
- final def !=(arg0: Any): Boolean
- Definition Classes
- AnyRef → Any
- final def ##: Int
- Definition Classes
- AnyRef → Any
- def +(other: String): String
- Implicit
- This member is added by an implicit conversion from ProductClassShape[E, C] toany2stringadd[ProductClassShape[E, C]] performed by method any2stringadd in scala.Predef.
- Definition Classes
- any2stringadd
- def ->[B](y: B): (ProductClassShape[E, C], B)
- Implicit
- This member is added by an implicit conversion from ProductClassShape[E, C] toArrowAssoc[ProductClassShape[E, C]] performed by method ArrowAssoc in scala.Predef.
- Definition Classes
- ArrowAssoc
- Annotations
- @inline()
- final def ==(arg0: Any): Boolean
- Definition Classes
- AnyRef → Any
- final def asInstanceOf[T0]: T0
- Definition Classes
- Any
- def buildParams(extract: (Any) => Unpacked): Packed
Build a packed representation containing QueryParameters that can extract data from the unpacked representation later.
Build a packed representation containing QueryParameters that can extract data from the unpacked representation later. This method is not available for shapes where Mixed and Unpacked are different types.
- Definition Classes
- ProductNodeShape → Shape
- def buildValue(elems: IndexedSeq[Any]): C
Build a record value represented by this Shape from its element values.
Build a record value represented by this Shape from its element values.
- Definition Classes
- ProductClassShape → ProductNodeShape
- implicit val classTag: ClassTag[E]
- Definition Classes
- MappedScalaProductShape → MappedProductShape
- def clone(): AnyRef
- Attributes
- protected[lang]
- Definition Classes
- AnyRef
- Annotations
- @throws(classOf[java.lang.CloneNotSupportedException]) @HotSpotIntrinsicCandidate() @native()
- def copy(s: Seq[Shape[_ <: ShapeLevel, _, _, _]]): ProductClassShape[E, C]
Create a copy of this Shape with new element Shapes.
Create a copy of this Shape with new element Shapes. This is used for packing Shapes recursively.
- Definition Classes
- ProductClassShape → ProductNodeShape
- def encodeRef(value: Any, path: Node): Any
Encode a reference into a value of this Shape.
Encode a reference into a value of this Shape. This method may not be available for shapes where Mixed and Packed are different types.
- Definition Classes
- ProductNodeShape → Shape
- def ensuring(cond: (ProductClassShape[E, C]) => Boolean, msg: => Any): ProductClassShape[E, C]
- Implicit
- This member is added by an implicit conversion from ProductClassShape[E, C] toEnsuring[ProductClassShape[E, C]] performed by method Ensuring in scala.Predef.
- Definition Classes
- Ensuring
- def ensuring(cond: (ProductClassShape[E, C]) => Boolean): ProductClassShape[E, C]
- Implicit
- This member is added by an implicit conversion from ProductClassShape[E, C] toEnsuring[ProductClassShape[E, C]] performed by method Ensuring in scala.Predef.
- Definition Classes
- Ensuring
- def ensuring(cond: Boolean, msg: => Any): ProductClassShape[E, C]
- Implicit
- This member is added by an implicit conversion from ProductClassShape[E, C] toEnsuring[ProductClassShape[E, C]] performed by method Ensuring in scala.Predef.
- Definition Classes
- Ensuring
- def ensuring(cond: Boolean): ProductClassShape[E, C]
- Implicit
- This member is added by an implicit conversion from ProductClassShape[E, C] toEnsuring[ProductClassShape[E, C]] performed by method Ensuring in scala.Predef.
- Definition Classes
- Ensuring
- final def eq(arg0: AnyRef): Boolean
- Definition Classes
- AnyRef
- def equals(arg0: AnyRef): Boolean
- Definition Classes
- AnyRef → Any
- final def getClass(): Class[_ <: AnyRef]
- Definition Classes
- AnyRef → Any
- Annotations
- @HotSpotIntrinsicCandidate() @native()
- def getElement(value: Product, idx: Int): Any
Get the element value from a record value at the specified index.
Get the element value from a record value at the specified index.
- Definition Classes
- MappedScalaProductShape → ProductNodeShape
- def getIterator(value: Product): Iterator[Any]
Get an Iterator of a record value's element values.
Get an Iterator of a record value's element values. The default implementation repeatedly calls
getElement
.- Definition Classes
- MappedScalaProductShape → ProductNodeShape
- def hashCode(): Int
- Definition Classes
- AnyRef → Any
- Annotations
- @HotSpotIntrinsicCandidate() @native()
- final def isInstanceOf[T0]: Boolean
- Definition Classes
- Any
- final def ne(arg0: AnyRef): Boolean
- Definition Classes
- AnyRef
- final def notify(): Unit
- Definition Classes
- AnyRef
- Annotations
- @HotSpotIntrinsicCandidate() @native()
- final def notifyAll(): Unit
- Definition Classes
- AnyRef
- Annotations
- @HotSpotIntrinsicCandidate() @native()
- def pack(value: Any): Packed
Convert a value of this Shape's (mixed) type to the fully packed type
Convert a value of this Shape's (mixed) type to the fully packed type
- Definition Classes
- ProductNodeShape → Shape
- def packedShape: Shape[FlatShapeLevel, Packed, Unpacked, Packed]
Return the fully packed Shape
Return the fully packed Shape
- Definition Classes
- ProductNodeShape → Shape
- val shapes: Seq[Shape[_ <: ShapeLevel, _, _, _]]
The Shapes for the product elements.
The Shapes for the product elements.
- Definition Classes
- ProductClassShape → ProductNodeShape
- final def synchronized[T0](arg0: => T0): T0
- Definition Classes
- AnyRef
- def toBase(v: Any): ProductWrapper
- Definition Classes
- MappedProductShape
- def toMapped(v: Any): E
- Definition Classes
- ProductClassShape → MappedProductShape
- def toNode(value: Any): TypeMapping
Return an AST Node representing a mixed value.
Return an AST Node representing a mixed value.
- Definition Classes
- MappedProductShape → ProductNodeShape → Shape
- def toString(): String
- Definition Classes
- ProductNodeShape → AnyRef → Any
- final def wait(arg0: Long, arg1: Int): Unit
- Definition Classes
- AnyRef
- Annotations
- @throws(classOf[java.lang.InterruptedException])
- final def wait(arg0: Long): Unit
- Definition Classes
- AnyRef
- Annotations
- @throws(classOf[java.lang.InterruptedException]) @native()
- final def wait(): Unit
- Definition Classes
- AnyRef
- Annotations
- @throws(classOf[java.lang.InterruptedException])
Deprecated Value Members
- def finalize(): Unit
- Attributes
- protected[lang]
- Definition Classes
- AnyRef
- Annotations
- @throws(classOf[java.lang.Throwable]) @Deprecated
- Deprecated
(Since version 9)
- def formatted(fmtstr: String): String
- Implicit
- This member is added by an implicit conversion from ProductClassShape[E, C] toStringFormat[ProductClassShape[E, C]] performed by method StringFormat in scala.Predef.
- Definition Classes
- StringFormat
- Annotations
- @deprecated @inline()
- Deprecated
(Since version 2.12.16) Use
formatString.format(value)
instead ofvalue.formatted(formatString)
, or use thef""
string interpolator. In Java 15 and later,formatted
resolves to the new method in String which has reversed parameters.
- def →[B](y: B): (ProductClassShape[E, C], B)
- Implicit
- This member is added by an implicit conversion from ProductClassShape[E, C] toArrowAssoc[ProductClassShape[E, C]] performed by method ArrowAssoc in scala.Predef.
- Definition Classes
- ArrowAssoc
- Annotations
- @deprecated
- Deprecated
(Since version 2.13.0) Use
->
instead. If you still wish to display it as one character, consider using a font with programming ligatures such as Fira Code.
edit this text on github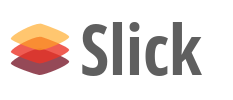
Scala Language-Integrated Connection Kit
This is the API documentation for the Slick database library. It should be used as an additional resource to the user manual.
Further documentation for Slick can be found on the documentation pages.
To the slick package list...