package jdbc
Contains the abstract JdbcProfile
and related code. This includes all JDBC-related code,
facilities for Plain SQL queries, and JDBC-specific profile components.
- Source
- package.scala
- Alphabetic
- By Inheritance
- jdbc
- AnyRef
- Any
- Hide All
- Show All
- Public
- Protected
Type Members
- final class ActionBasedSQLInterpolation extends AnyVal
- class BaseResultConverter[T] extends ResultConverter[ResultSet, PreparedStatement, ResultSet, T]
Specialized JDBC ResultConverter for non-
Option
values. - class ConnectionPreparer extends (Connection) => Unit
Set parameters on a new Connection.
Set parameters on a new Connection. This is used by DataSourceJdbcDataSource.
- trait DB2Profile extends JdbcProfile with MultipleRowsPerStatementSupport
Slick profile for IBM DB2 UDB.
Slick profile for IBM DB2 UDB.
This profile implements slick.jdbc.JdbcProfile without the following capabilities:
- slick.relational.RelationalCapabilities.reverse: This String function is not available in DB2.
- slick.jdbc.JdbcCapabilities.insertOrUpdate: InsertOrUpdate operations are emulated on the client side if generated keys should be returned. Otherwise the operation is performed natively on the server side.
- slick.jdbc.JdbcCapabilities.booleanMetaData: DB2 doesn't have booleans, so Slick maps to SMALLINT instead. Other JDBC drivers like MySQL map TINYINT(1) back to a Scala Boolean. DB2 maps SMALLINT to an Integer and that's how it shows up in the JDBC meta data, thus the original type is lost.
- slick.jdbc.JdbcCapabilities.supportsByte: DB2 does not have a BYTE type.
Note: The DB2 JDBC driver has problems with quoted identifiers. Columns which are returned from inserts must not require quoted names (in particular, they must not contain lower-case characters or be equal to a reserved word), otherwise a bug in the DB2 JDBC driver triggers a SQL Error -206 (SQLState 42703).
- class DataSourceJdbcDataSource extends JdbcDataSource
A JdbcDataSource for a
DataSource
- class DatabaseUrlDataSource extends DriverDataSource
A DataSource that wraps the DriverManager API.
A DataSource that wraps the DriverManager API. It can be configured as a Java Bean and used both stand-alone and as a source for a connection pool. This implementation is design specifically to handle a non-JDBC Database URL in the format defined by the libpq standard.
- class DefaultingResultConverter[T] extends ResultConverter[ResultSet, PreparedStatement, ResultSet, T]
Specialized JDBC ResultConverter for handling non-
Option
values with a default.Specialized JDBC ResultConverter for handling non-
Option
values with a default. A (possibly specialized) function for the default value is used to translate SQLNULL
values. - trait DerbyProfile extends JdbcProfile with MultipleRowsPerStatementSupport
Slick profile for Derby/JavaDB.
Slick profile for Derby/JavaDB.
This profile implements slick.jdbc.JdbcProfile without the following capabilities:
- slick.relational.RelationalCapabilities.functionDatabase:
Functions.database
is not available in Derby. Slick will return an empty string instead. - slick.relational.RelationalCapabilities.replace, slick.relational.RelationalCapabilities.reverse: These String functions are not available in Derby.
- slick.relational.RelationalCapabilities.pagingNested: See DERBY-5911.
- slick.jdbc.JdbcCapabilities.returnInsertOther: When returning columns from an INSERT operation, only a single column may be specified which must be the table's AutoInc column.
- slick.sql.SqlCapabilities.sequenceCurr:
Sequence.curr
to get the current value of a sequence is not supported by Derby. Trying to generate SQL code which uses this feature throws a SlickException. - slick.sql.SqlCapabilities.sequenceCycle: Sequence cycling is supported but does not conform to SQL:2008 semantics. Derby cycles back to the START value instead of MINVALUE or MAXVALUE.
- slick.relational.RelationalCapabilities.zip:
Ordered sub-queries and window functions with orderings are currently
not supported by Derby. These are required by
zip
andzipWithIndex
. Trying to generate SQL code which uses this feature causes the DB to throw an exception. We do not prevent these queries from being generated because we expect future Derby versions to support them with the standard SQL:2003 syntax (see http://wiki.apache.org/db-derby/OLAPRowNumber). - slick.relational.RelationalCapabilities.joinFull: Full outer joins are emulated because there is not native support for them.
- slick.jdbc.JdbcCapabilities.insertOrUpdate: InsertOrUpdate operations are emulated on the client side because there is no native support for them (but there is work in progress: see DERBY-3155).
- slick.jdbc.JdbcCapabilities.booleanMetaData: Derby <= 10.6 doesn't have booleans, so Slick maps to SMALLINT instead. Other jdbc drivers like MySQL map TINYINT(1) back to a Scala Boolean. Derby maps SMALLINT to an Integer and that's how it shows up in the jdbc meta data, thus the original type is lost.
- slick.jdbc.JdbcCapabilities.supportsByte: Derby doesn't have a corresponding type for Byte. SMALLINT is used instead and mapped to Short in the Slick model.
- slick.relational.RelationalCapabilities.repeat: There's not builtin string function repeat in Derby. https://db.apache.org/derby/docs/10.10/ref/rrefsqlj29026.html
- slick.jdbc.JdbcCapabilities.returnMultipleInsertKey: Derby's driver is unable to properly return the generated key for insert statements with multiple values. (DERBY-3609)
- slick.relational.RelationalCapabilities.functionDatabase:
- class DriverDataSource extends DataSource with Closeable with Logging
A DataSource that wraps the DriverManager API.
A DataSource that wraps the DriverManager API. It can be configured as a Java Bean and used both stand-alone and as a source for a connection pool.
- trait GetResult[+T] extends (PositionedResult) => T
Basic conversions for extracting values from PositionedResults.
- class GetTupleResult[+T <: Product] extends GetResult[T]
GetResult for tuple types.
- trait H2Profile extends JdbcProfile with MultipleRowsPerStatementSupport
Slick profile for H2.
Slick profile for H2.
This profile implements slick.jdbc.JdbcProfile without the following capabilities:
- slick.relational.RelationalCapabilities.reverse: This String function is not available in H2.
- slick.sql.SqlCapabilities.sequenceMin, slick.sql.SqlCapabilities.sequenceMax, slick.sql.SqlCapabilities.sequenceCycle: H2 does not support MINVALUE, MAXVALUE and CYCLE
- slick.jdbc.JdbcCapabilities.returnInsertOther: When returning columns from an INSERT operation, only a single column may be specified which must be the table's AutoInc column.
- slick.relational.RelationalCapabilities.joinFull: Full outer joins are emulated because there is not native support for them.
- slick.jdbc.JdbcCapabilities.insertOrUpdate:
InsertOrUpdate operations are emulated on the client side if the
data to insert contains an
AutoInc
fields. Otherwise the operation is performed natively on the server side.
- trait HsqldbProfile extends JdbcProfile with MultipleRowsPerStatementSupport
Slick profile for HyperSQL (starting with version 2.0).
Slick profile for HyperSQL (starting with version 2.0).
This profile implements the slick.jdbc.JdbcProfile without the following capabilities:
- slick.sql.SqlCapabilities.sequenceCurr:
Sequence.curr
to get the current value of a sequence is not supported by Hsqldb. Trying to generate SQL code which uses this feature throws a SlickException. - slick.jdbc.JdbcCapabilities.insertOrUpdate: InsertOrUpdate operations are emulated on the client side if generated keys should be returned. Otherwise the operation is performed natively on the server side.
- slick.sql.SqlCapabilities.sequenceCurr:
- class InsertBuilderResult extends AnyRef
- trait Invoker[+R] extends AnyRef
Base trait for all statement invokers of result element type R.
- class IsDefinedResultConverter[T] extends ResultConverter[ResultSet, PreparedStatement, ResultSet, Boolean]
Specialized JDBC ResultConverter for handling
isDefined
checks forOption
values. - trait JdbcActionComponent extends SqlActionComponent
- trait JdbcBackend extends RelationalBackend
A JDBC-based database back-end that is used by slick.jdbc.JdbcProfile.
- trait JdbcDataSource extends Closeable
A
JdbcDataSource
provides a way to create aConnection
object for a database.A
JdbcDataSource
provides a way to create aConnection
object for a database. It is similar to ajavax.sql.DataSource
but simpler. Unlike JdbcBackend.JdbcDatabaseDef it is not a part of the backend cake. This trait defines the SPI for 3rd-party connection pool support. - trait JdbcDataSourceFactory extends AnyRef
Create a JdbcDataSource from a
Config
object and an optional JDBCDriver
.Create a JdbcDataSource from a
Config
object and an optional JDBCDriver
. This is used with the "connectionPool" configuration option in JdbcBackend.DatabaseFactoryDef.forConfig. - trait JdbcInvokerComponent extends AnyRef
- trait JdbcMappingCompilerComponent extends AnyRef
JDBC profile component which contains the mapping compiler and insert compiler
- class JdbcModelBuilder extends Logging
Build a Slick model from introspecting the JDBC metadata.
Build a Slick model from introspecting the JDBC metadata.
In most cases you are better off transforming the generated model instead of overriding functionality here. It is only useful if you need easy access to the JDBC metadata in order to influence how the model is generated. A good use case would be interpreting column types or default values that Slick doesn't understand out of the box. If you just want to remove or hard code some default values, transform the resulting model instead.
The tight coupling can easily lead to source code incompatibilities in future versions. Avoid hooking in here if you don't have to.
- trait JdbcModelComponent extends AnyRef
- trait JdbcProfile extends SqlProfile with JdbcActionComponent with JdbcInvokerComponent with JdbcTypesComponent with JdbcModelComponent with JdbcStatementBuilderComponent with JdbcMappingCompilerComponent
Abstract profile for accessing SQL databases via JDBC.
- trait JdbcStatementBuilderComponent extends AnyRef
- trait JdbcType[T] extends BaseTypedType[T]
A JdbcType object represents a Scala type that can be used as a column type in the database.
A JdbcType object represents a Scala type that can be used as a column type in the database. Implicit JdbcTypes for the standard types are provided by the profile.
- trait JdbcTypesComponent extends RelationalTypesComponent
- class LoggingPreparedStatement extends LoggingStatement with PreparedStatement
A wrapper for
java.sql.PreparedStatement
that logs statements, parameters and benchmark results to the appropriate JdbcBackend loggers. - class LoggingStatement extends Statement
A wrapper for
java.sql.Statement
that logs statements and benchmark results to the appropriate JdbcBackend loggers. - trait MySQLProfile extends JdbcProfile with MultipleRowsPerStatementSupport
Slick profile for MySQL.
Slick profile for MySQL.
This profile implements slick.jdbc.JdbcProfile without the following capabilities:
- slick.jdbc.JdbcCapabilities.returnInsertOther: When returning columns from an INSERT operation, only a single column may be specified which must be the table's AutoInc column.
- slick.sql.SqlCapabilities.sequenceLimited: Non-cyclic sequence may not have an upper limit.
- slick.relational.RelationalCapabilities.joinFull: Full outer joins are emulated because there is not native support for them.
- slick.jdbc.JdbcCapabilities.nullableNoDefault: Nullable columns always have NULL as a default according to the SQL standard. Consequently MySQL treats no specifying a default value just as specifying NULL and reports NULL as the default value. Some other dbms treat queries with no default as NULL default, but distinguish NULL from no default value in the meta data.
Sequences are supported through an emulation which requires the schema to be created by Slick. You can also use an existing schema with your own sequence emulation if you provide for each sequence s a pair of functions
s_nextval
ands_currval
.The default type for strings of unlimited length is "TEXT", falling back to "VARCHAR(254)" if a
Default
orPrimaryKey
column option is set. This can be changed by overridingslick.jdbc.MySQLProfile.defaultStringType
in application.conf.Note: Slick 3.2 also checks the old config path "slick.driver.MySQL" that was used by Slick 3.0 and 3.1, and logs a warning if anything is found there. Values from the old path are *not* used anymore. This deprecation warning will be removed in a future version.
- class OptionResultConverter[T] extends ResultConverter[ResultSet, PreparedStatement, ResultSet, Option[T]]
Specialized JDBC ResultConverter for handling values of type
Option[T]
.Specialized JDBC ResultConverter for handling values of type
Option[T]
. Boxing is avoided when the result isNone
. - trait OracleProfile extends JdbcProfile
Slick profile for Oracle.
Slick profile for Oracle.
This profile implements slick.jdbc.JdbcProfile without the following capabilities:
- slick.relational.RelationalCapabilities.foreignKeyActions: Foreign key actions Cascade, SetNull and NoAction are directly supported for onDelete. Restrict and SetDefault are ignored (i.e. equals to NoAction). No onUpdate actions are supported but specifying Cascade adds the option INITIALLY DEFERRED to the foreign key constraint, thus allowing you to perform the cascading update manually before committing the current transaction. Other onUpdate actions are ignored.
- slick.jdbc.JdbcCapabilities.insertOrUpdate: InsertOrUpdate operations are emulated on the client side if generated keys should be returned. Otherwise the operation is performed natively on the server side.
- slick.jdbc.JdbcCapabilities.booleanMetaData: Oracle doesn't have booleans, so Slick maps to CHAR instead and that's how it appears in model and generated code.
- slick.jdbc.JdbcCapabilities.distinguishesIntTypes: Oracle doesn't distinguish integer types and Slick uses NUMBER, which is always mapped back to BigDecimal in model and generated code.
- slick.jdbc.JdbcCapabilities.supportsByte: Oracle does not have a BYTE type.
- slick.jdbc.JdbcCapabilities.returnMultipleInsertKey: Oracle returns the last generated key only.
- slick.jdbc.JdbcCapabilities.insertMultipleRowsWithSingleStatement: Oracle doesn't support this feature directly. There are several alternative ways, but the library doesn't support them, so far.
Note: The Oracle JDBC driver has problems with quoted identifiers. Columns which are returned from inserts must not require quoted names (in particular, they must not contain lower-case characters or be equal to a reserved word), otherwise a bug in the Oracle JDBC driver triggers an ORA-00904 error. The same issue arises when trying to update such a column in a mutable result set.
Updating Blob values in updatable result sets is not supported.
- class PositionedParameters extends AnyRef
A wrapper for a JDBC
PreparedStatement
which allows inceremental setting of parameters without having to sepcify the column index each time. - abstract class PositionedResult extends Closeable
A database result positioned at a row and column.
- abstract class PositionedResultIterator[+T] extends ReadAheadIterator[T] with CloseableIterator[T]
An CloseableIterator for a PositionedResult.
- trait PostgresProfile extends JdbcProfile with MultipleRowsPerStatementSupport
Slick profile for PostgreSQL.
Slick profile for PostgreSQL.
This profile implements slick.jdbc.JdbcProfile without the following capabilities:
- slick.jdbc.JdbcCapabilities.insertOrUpdate:
InsertOrUpdate operations are emulated on the server side with a single
JDBC statement executing multiple server-side statements in a transaction.
This is faster than a client-side emulation but may still fail due to
concurrent updates. InsertOrUpdate operations with
returning
are emulated on the client side. - slick.jdbc.JdbcCapabilities.nullableNoDefault: Nullable columns always have NULL as a default according to the SQL standard. Consequently Postgres treats no specifying a default value just as specifying NULL and reports NULL as the default value. Some other dbms treat queries with no default as NULL default, but distinguish NULL from no default value in the meta data.
- slick.jdbc.JdbcCapabilities.supportsByte: Postgres doesn't have a corresponding type for Byte. SMALLINT is used instead and mapped to Short in the Slick model.
Notes:
- slick.relational.RelationalCapabilities.typeBlob:
The default implementation of the
Blob
type uses the database typelo
and the stored procedurelo_manage
, both of which are provided by the "lo" extension in PostgreSQL.
- slick.jdbc.JdbcCapabilities.insertOrUpdate:
InsertOrUpdate operations are emulated on the server side with a single
JDBC statement executing multiple server-side statements in a transaction.
This is faster than a client-side emulation but may still fail due to
concurrent updates. InsertOrUpdate operations with
- class ProtectGroupBy extends Phase
Ensure that every expression in a GroupBy's "by" clause contains a reference to a proper source field.
Ensure that every expression in a GroupBy's "by" clause contains a reference to a proper source field. If this is not the case, wrap the source in a Subquery boundary.
- sealed abstract class ResultSetConcurrency extends AnyRef
Represents a result set concurrency mode.
- sealed abstract class ResultSetHoldability extends AnyRef
Represents a result set holdability mode .
- abstract class ResultSetInvoker[+R] extends Invoker[R]
An invoker which calls a function to retrieve a ResultSet.
An invoker which calls a function to retrieve a ResultSet. This can be used for reading information from a java.sql.DatabaseMetaData object which has many methods that return ResultSets.
For convenience, if the function returns null, this is treated like an empty ResultSet.
- trait ResultSetMutator[T] extends AnyRef
- sealed abstract class ResultSetType extends AnyRef
Represents a result set type.
- sealed trait RowsPerStatement extends AnyRef
- case class SQLActionBuilder(sql: String, setParameter: SetParameter[Unit]) extends Product with Serializable
- trait SQLServerProfile extends JdbcProfile with MultipleRowsPerStatementSupport
Slick profile for Microsoft SQL Server.
Slick profile for Microsoft SQL Server.
This profile implements slick.jdbc.JdbcProfile without the following capabilities:
- slick.jdbc.JdbcCapabilities.returnInsertOther: When returning columns from an INSERT operation, only a single column may be specified which must be the table's AutoInc column.
- slick.sql.SqlCapabilities.sequence: Sequences are not supported because SQLServer does not have this feature.
- slick.jdbc.JdbcCapabilities.forceInsert: Inserting explicit values into AutoInc columns with forceInsert operations is not supported.
- slick.jdbc.JdbcCapabilities.createModel: Reading the database schema is not supported.
- slick.jdbc.JdbcCapabilities.insertOrUpdate: InsertOrUpdate operations are emulated on the client side if generated keys should be returned. Otherwise the operation is performed natively on the server side.
- slick.jdbc.JdbcCapabilities.supportsByte: SQL Server's TINYINT is unsigned. It doesn't have a signed Byte-like type. Slick maps Byte to SMALLINT instead and that's how it shows up in model and code-generation.
The default type for strings of unlimited length is "VARCHAR(MAX)", falling back to "VARCHAR(254)" if a
PrimaryKey
column option is set. This can be changed by overridingslick.jdbc.SQLServerProfile.defaultStringType
in application.conf. - trait SQLiteProfile extends JdbcProfile with MultipleRowsPerStatementSupport
Slick profile for SQLite.
Slick profile for SQLite.
This profile implements slick.jdbc.JdbcProfile without the following capabilities:
- slick.relational.RelationalCapabilities.functionDatabase,
slick.relational.RelationalCapabilities.functionUser:
Functions.user
andFunctions.database
are not available in SQLite. Slick will return empty strings for both. - slick.relational.RelationalCapabilities.joinFull, slick.relational.RelationalCapabilities.joinRight: Right and full outer joins are emulated because there is not native support for them.
- slick.jdbc.JdbcCapabilities.mutable: SQLite does not allow mutation of result sets. All cursors are read-only.
- slick.sql.SqlCapabilities.sequence: Sequences are not supported by SQLite.
- slick.jdbc.JdbcCapabilities.returnInsertOther: When returning columns from an INSERT operation, only a single column may be specified which must be the table's AutoInc column.
- slick.relational.RelationalCapabilities.typeBigDecimal: SQLite does not support a decimal type.
- slick.relational.RelationalCapabilities.typeBlob: Blobs are
not supported by the SQLite JDBC driver (but binary data in the form of
Array[Byte]
is). - slick.relational.RelationalCapabilities.zip:
Row numbers (required by
zip
andzipWithIndex
) are not supported. Trying to generate SQL code which uses this feature throws a SlickException. - slick.jdbc.JdbcCapabilities.insertOrUpdate:
InsertOrUpdate operations are emulated on the client side if the
data to insert contains an
AutoInc
field. Otherwise the operation is performed natively on the server side. - slick.jdbc.JdbcCapabilities.defaultValueMetaData: The stable xerial sqlite-jdbc driver 3.7.2 does not return default values for columns in the DatabaseMetaData. Consequently they also do not appear in Slick's model. This has been fixed in sqlite-jdbc, but the only released version that contains the fix is milestone 3.7.15-M1. You can use it instead of the stable 3.7.2 in order to get default values with SQLite. Also see https://code.google.com/p/sqlite-jdbc/issues/detail?id=27
- slick.jdbc.JdbcCapabilities.booleanMetaData: SQLite doesn't have booleans, so Slick maps to INTEGER instead. Other jdbc drivers like MySQL map TINYINT(1) back to a Scala Boolean. SQLite maps INTEGER to an Integer and that's how it shows up in the jdbc meta data, thus the original type is lost.
- slick.jdbc.JdbcCapabilities.distinguishesIntTypes: SQLite does not distinguish integer types and maps them all to Int in the meta data.
- slick.jdbc.JdbcCapabilities.supportsByte: SQLite does not distinguish integer types and maps them all to Int in the meta data.
- slick.jdbc.JdbcCapabilities.returnMultipleInsertKey: SQLite returns the last generated key only.
- slick.relational.RelationalCapabilities.functionDatabase,
slick.relational.RelationalCapabilities.functionUser:
- trait SetParameter[-T] extends (T, PositionedParameters) => Unit
Basic conversions for setting parameters in PositionedParameters.
- class SetTupleParameter[-T <: Product] extends SetParameter[T]
SetParameter for tuple types.
- case class SimpleJdbcAction[+R](f: (JdbcActionContext) => R) extends SynchronousDatabaseAction[R, NoStream, JdbcActionContext, JdbcStreamingActionContext, All] with Product with Serializable
A non-streaming Action that wraps a JDBC call.
- abstract class StatementInvoker[+R] extends Invoker[R]
An invoker which executes an SQL statement through JDBC.
- trait StreamingInvokerAction[R, T, -E <: Effect] extends SynchronousDatabaseAction[R, Streaming[T], JdbcActionContext, JdbcStreamingActionContext, E] with FixedSqlStreamingAction[R, T, E]
A streaming Action that wraps an Invoker.
A streaming Action that wraps an Invoker. It is used for the Lifted Embedding, Direct Embedding, Plain SQL queries, and JDBC metadata.
- sealed abstract class TransactionIsolation extends AnyRef
Represents a transaction isolation level.
- class TypedParameter[T] extends AnyRef
Value Members
- object DB2Profile extends DB2Profile
- object DataSourceJdbcDataSource extends JdbcDataSourceFactory
- object DerbyProfile extends DerbyProfile
- object GetResult
- object H2Profile extends H2Profile
- object HsqldbProfile extends HsqldbProfile
- object JdbcActionComponent
- object JdbcBackend extends JdbcBackend
- object JdbcCapabilities
Capabilities for slick.jdbc.JdbcProfile.
- object JdbcDataSource extends Logging
- object JdbcTypesComponent
- object MySQLProfile extends MySQLProfile
- object OracleProfile extends OracleProfile with OneRowPerStatementOnly
- object PGUtils
- object PostgresProfile extends PostgresProfile
- object ResultSetAction
- object ResultSetConcurrency
- object ResultSetHoldability
- object ResultSetInvoker
- object ResultSetType
- object RowsPerStatement
- object SQLActionBuilder extends Serializable
- object SQLServerProfile extends SQLServerProfile
- object SQLiteProfile extends SQLiteProfile
- object SetParameter
- object SpecializedJdbcResultConverter
Factory methods for JdbcResultConverters which are manually specialized on the underlying JdbcType.
Factory methods for JdbcResultConverters which are manually specialized on the underlying JdbcType. A generic implementation of this factory still provides allocation free call paths but performs almost 100% slower in the fast path benchmark.
- object TimestamptzConverter
Converts between
TIMESTAMPTZ
and java.time times and back.Converts between
TIMESTAMPTZ
and java.time times and back. Oracle jar not on path at compile time (but must be a run time) Use reflection to get access toTIMESTAMPTZ
class - object TransactionIsolation
- object TypedParameter
edit this text on github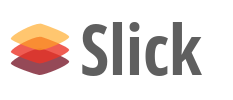
Scala Language-Integrated Connection Kit
This is the API documentation for the Slick database library. It should be used as an additional resource to the user manual.
Further documentation for Slick can be found on the documentation pages.
To the slick package list...